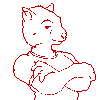 |
Serial Wombat 4A and 4B Firmware
|
Go to the documentation of this file.
33 #define BYTES_PER_PIN_REGISTER 20
35 #define BYTES_PER_PIN_REGISTER 64
36 #define BYTES_AVAIALABLE_OUTPUT_PULSE 50
37 #define BYTES_AVAILABLE_INPUT_DMA (BYTES_PER_PIN_REGISTER - 5)
60 uint8_t
bytes[BYTES_AVAIALABLE_OUTPUT_PULSE];
63 uint16_t highRemaining;
64 uint16_t lowRemaining;
73 uint8_t
bytes[BYTES_AVAILABLE_INPUT_DMA];
86 #define BUILD_BUG_ON(condition) ((void)sizeof(char[1 - 2*!!(condition)]))
92 #define USE_BUFFERED_PIN_REGISTER
95 #ifdef USE_BUFFERED_PIN_REGISTER
99 #define CurrentPinRegister (&pinRegisterBuffer)
107 void SetBuffer(uint8_t pin, uint16_t value);
109 void SetMode(uint8_t pin, uint8_t mode);
uint8_t bytes[BYTES_PER_PIN_REGISTER]
Definition: pinRegisters.h:41
pinRegister_t PinUpdateRegisters[NUMBER_OF_TOTAL_PINS]
Definition: pinRegisters.c:28
void CopyFromPinBufferToArray(void)
Definition: pinRegisters.c:37
uint16_t buffer
A 16 bit 'public' variable which is in the same location for every pin.
Definition: pinRegisters.h:51
#define BYTES_PER_PIN_REGISTER
Definition: pinRegisters.h:33
void SetBuffer(uint8_t pin, uint16_t value)
Definition: pinRegisters.c:46
uint8_t GetMode(uint8_t pin)
Definition: pinRegisters.c:102
union _pin_register_t pinRegister_t
pinRegister_t pinRegisterBuffer
Definition: main.c:62
#define NUMBER_OF_TOTAL_PINS
Definition: deviceSpecific.h:36
Definition: pinRegisters.h:40
uint8_t mode
The mode of the current pin.
Definition: pinRegisters.h:55
void SetMode(uint8_t pin, uint8_t mode)
Definition: pinRegisters.c:94
uint16_t uwords[BYTES_PER_PIN_REGISTER/2]
Definition: pinRegisters.h:42
#define CurrentPinRegister
Definition: pinRegisters.h:99
uint16_t pinUwords[(BYTES_PER_PIN_REGISTER/2) - 2]
Definition: pinRegisters.h:47
void CopyFromArrayToPinBuffer(void)
Definition: pinRegisters.c:32
uint16_t GetBuffer(uint8_t pin)
Definition: pinRegisters.c:54
A generic descriptor, typically used to access the buffer and mode variables.
Definition: pinRegisters.h:46